This post will cover everything that you need to know in practice in order to handle all sorts of file upload scenarios in an Angular application.
We are going to learn how to build a fully functional Angular file upload component, that requires a file of a given extension to be uploaded and sends the file to a backend via an HTTP POST call.
This custom component is going to have an upload loading indicator, and it's going to support upload cancelation as well. We are going to give also an example (in Node) of how to handle the file in the backend.
Table Of Contents
In this post, we will cover the following topics:
- How to upload files in a browser
- Building the user interface of a file upload component
- Selecting a file from the file system using a file upload dialog
- Uploading a file to the backend using the Angular HTTP Client
- How to display a file upload progress indicator
- How to cancel an ongoing file upload
- Handling the uploaded file on a Node backend
- How to upload multiple files
- Summary
So without further ado, let's get started learning how to build an Angular file upload component!
How to Upload Files in a Browser
In order to build an Angular file upload component, we need to first understand how to upload files in plain HTML and Javascript only, and take it from there.
The key ingredient for uploading files in a browser is a plain HTML input of type file:
This input will allow the user to open a browser file selection dialog and select one or more files (by default, only one file). Here is what this input looks like:
With this file input box, you can select a file from your file system, and then with a bit of Javascript, you can already send it to a backend.
Why don't we see file inputs very often?
The problem with this plain file input is that by default it's very hard to style. Some styles applied to it can't be changed, and we can't even change the text on the button!
This is default browser behavior that can't be changed, and that is why we usually don't see this plain file input on all the interfaces that we use daily, like Gmail, etc.
Because this file input is impossible to style properly, the most common option is to actually never show it to the end-user, as we will see.
How does the input of type file work?
When the user chooses a file using the file upload dialog, an event of type
change
will be emitted. This event will then contain the list of files that the user selected on the target.files
property.
Here is the output that we see on the console after the user selects a file:
When the change
event gets triggered, the file is not automatically uploaded to the backend by the browser. Instead, we will need to trigger an HTTP request ourselves, in response to the change
event.
Now that we know how all the standard file upload browser functionality works, let's see how can we build a nice Angular component to encapsulate it.
Building the User Interface of a File Upload Component
Because a plain input of type file is impossible to style properly, what we end up doing is hiding it from the user, and then building an alternative file upload UI that uses the file input behind the scenes.
Here is what the template of an initial file upload component could look like:
This user interface is split up into two separate parts. On top, we have a plain file input, that is used to open the file upload dialog and handle the change
event.
This plain input text is hidden from the user, as we can see in the component CSS:
Below the hidden file input, we have the file-upload
container div, which contains the actual UI that the user will see on the screen.
As an example, we have built this UI with Angular Material components, but of course, the alternative file upload UI could take any form that you like.
This UI could be a dialog, a drag and drop zone, or like in the case of our component, just a styled button:
Notice in the component template how the upload blue button and the invisible file input are linked. When the user clicks on the blue button, a click handler triggers the file input via fileUpload.click()
.
The user will then choose a file from the file upload dialog, and the change
event will be triggered and handled by onFileSelected()
.
Uploading a file to the backend using the Angular HTTP Client
Let's now have a look at our component class and the implementation of
onFileSelected()
:
Here is what is going in this component:
- we are getting a reference to the files that the user selected by accessing the
event.target.files
property. - we then build a form payload by using the
FormData
API. This is a standard browser API, it's not Angular-specific. - we then use the Angular HTTP client to create an HTTP request and send the file to the backend
At this point, we would already have a working file upload component. But we want to take this component one step further. We want to be able to display a progress indicator to the user, and also support upload cancelation.
How to Display a File Upload Progress Indicator
We are going to add a few more elements to the UI of our file upload component. Here is the final version of the file upload component template:
The two main elements that we have added to the UI are:
- An Angular Material progress bar, which is visible only while the file upload is still in progress.
- A cancel upload button, also only visible when an upload is still ongoing
How to know how much of the file has been uploaded?
The way that we implement the progress indicator, is by using the reportProgress
feature of the Angular HTTP client.
With this feature, we can get notified of the progress of a file upload via multiple events emitted by the HTTP Observable.
To see it in action, let's have a look at the final version of the file upload component class, with all its features implemented:
As we can see, we have set the reportProgress
property to true in our HTTP call, and we have also set the observe
property to the value events
.
This means that, as the POST call continues, we are going to receive event objects reporting the progress of the HTTP request.
These events will be emitted as values of the http$
Observable, and come in different types:
- events of type
UploadProgress
report the percentage of the file that has already been uploaded - events of types
Response
report that the upload has been completed
Using the events of type UploadProgress
, we are saving the ongoing upload percentage in a member variable uploadProgress
, which we then use to update the value of the progress indicator bar.
When the upload either completes or fails, we need to hide the progress bar from the user.
We can make sure that we do so by using the RxJs finalize
operator, which is going to call the reset()
method in both cases: upload success or failure.
How to Cancel an Ongoing File Upload
In order to support file upload cancellation, all we have to is keep a reference to the RxJs Subscription object that we get when the http$
Observable gets subscribed to.
In our component, we store this subscription object in the uploadSub
member variable.
While the upload is still in progress, the user might decide to cancel it by clicking on the cancel button. Then the cancelUpload()
upload method is going to get triggered and the HTTP request can be canceled by unsubscribing from the uploadSub
subscription.
This unsubscription will trigger the immediate cancelation of the ongoing file upload.
How to accept only files of a certain type
In the final version of our file upload component, we can require the user to upload a file of a certain type, by using the requiredFileType
property:
This property is then passed directly to the accept
property of the file input in the file upload template, forcing the user to select a png file from the file upload dialog.
How to Upload Multiple Files
By default, the browser file selection dialog will allow the user to select only one file for upload.
But using the multiple
property, we can allow the user to select multiple files instead:
Notice that this would need a completely different UI than the one that we have built. A styled upload button with a progress indicator only works well for the upload of a single file.
For a multi-file upload scenario, there are a variety of UIs that could be built: a floating dialog with the upload progress of all files, etc.
Handling the uploaded file on a Node backend
The way that you handle the uploaded file in your backend depends on the technology that you use, but let's give a quick example of how to do it if using Node and the Express framework.
We need to first install the express-fileupload
package. We can then add this package as a middleware in our Express application:
From here, all we have to do is define an Express route to handle the file upload requests:
Summary
The best way to handle file upload in Angular is to build one or more custom components, depending on the supported upload scenarios.
A file upload component needs to contain internally an HTML input of type file, that allows the user to select one or more files from the file system.
This file input should be hidden from the user as it's not styleable and replaced by a more user-friendly UI.
Using the file input in the background, we can get a reference to the file via the change
event, which we can then use to build an HTTP request and send the file to the backend.
I hope that you have enjoyed this post, if you would like to learn a lot more about Angular, we recommend checking the Angular Core Deep Dive course, where we will cover all of the advanced Angular features in detail.
Also, if you have some questions or comments please let me know in the comments below and I will get back to you.
To get notified of upcoming posts on Angular, I invite you to subscribe to our newsletter:
And if you are just getting started learning Angular, have a look at the Angular for Beginners Course:
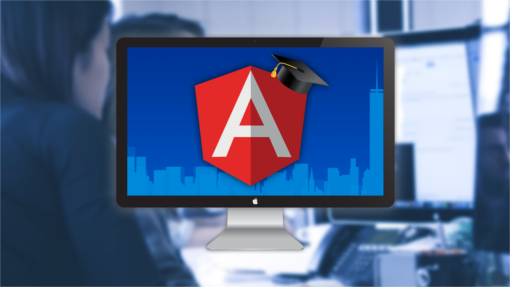