In this post, we will learn how the default Angular styling mechanism (Emulated Encapsulation) works under the hood, and we will also cover the Sass support of the Angular CLI, and some best practices for how to leverage the many Sass features available.
We will talk about when to use each feature and why, talk about the benefits of component style isolation and also cover how to debug our styles if something is not working.
This is the second post of a two-part series in Angular Component Styling, if you are looking to learn about ngClass and ngStyle, have a look at part one:
Angular ngClass and ngStyle: The Complete Guide
Table Of Contents
This post will cover the following topics:
- Angular Style Isolation - How does it work?
- Debugging Angular Styles
- The
:host
modifier, when to use it and why - The
:host-context
modifier, common use cases for theming - The
/deep/
,::ng-deep
or>>>
modifiers (upcoming deprecation) - How to style content that was projected using
ng-content
- Angular CLI CSS Preprocessors support - Sass, Less and Stylus
- How can we use Sass to improve our styles
- Summary
In order to cover each feature, we will be adding the multiple examples to this small Angular CLI sample application, that will use as external styles a Bootstrap default theme.
Why Style Isolation?
So without further ado, let's get started with our Angular Style Isolation deep dive. The first question that comes to mind is, why would we want to isolate the styles of our components? There are a couple of reasons for that, and one key reason is CSS maintainability.
As we develop a component style suite for an application, we tend to run into situations where the styles from one feature start interfering with the styles of another feature.
This is because browsers do not have yet widespread support for style isolation, where we can constrain one style to be applied only to one particular part of the page.
If we are not careful and systematically organize our styles to prevent this issue (for example using a methodology like SMACSS), we will quickly run into CSS maintenance issues.
Wouldn't it be great to be able to style our components with just short, simple and easy to read selectors, without having to worry about all the scenarios where those styles could be accidentally overridden?
Another benefit of style isolation
Here is another scenario: how many times did we try to use a third-party component, add it to our application just to find out that the component is completely broken due to styling issues?
Style isolation would allow us to ship our components knowing that the styles of the component will (most likely) not be overridden by other styles in target applications.
This makes the component effectively much more reusable, because the component will now in most cases simply just work, styles included.
Angular View Encapsulation brings us all of these advantages, so let's learn how it works!
A Demo of Angular Emulated Encapsulation
In this section, we will see how Angular component styling works under the hood, as this is the best way to understand it. This will also allow us to debug the mechanism if needed.
This is a video demonstration of the default mechanism in action:
In order to benefit from the default view encapsulation mechanism of Angular, all we need to do is to add our CSS classes to an external CSS file:
But then, instead of adding this file to our index.html
as a link
tag, we will instead associate it with our component using the styleUrls
property:
The color red would then be applied to this button, as expected. But what if now we have another button, for example directly at the level of our index.html
?
If you didn't know that there was some sort of style isolation mechanism in action, you might be surprised to find out that this button does NOT get a red background!
So what is going on here? Let's see how this mechanism works, because knowing that is what is going to allow us to debug it if needed.
How does Angular Style Isolation work? Emulated View Encapsulation
To better understand how default view encapsulation works, let's see what the app-root
custom HTML element will look like at runtime:
Several things are going on at the level of the runtime HTML:
- a strange looking property was added to the
ap-root
custom element: the_nghost-c0
property - Each of the HTML elements inside the application root component got another strange looking but different property:
_ngcontent-c0
What are these properties?
So how do these properties work? To better understand these properties and how they enable style isolation, we are going to create a second separate component, that just contains a button with the blue color.
For simplicity, we will define the styles for this component inline next to the template:
And using this newly defined component, we are going to add it to the template of the application root component:
Try to guess at this stage what the HTML at runtime would look like, and what happened to those strangely named properties!
The host element and template element style isolation properties
With this second component in place, let's have a second look at the HTML. The way that these two properties work will now become much more apparent:
Notice the blue-button
element, we have now a new host property called _nghost-c1
.
The blue-button
element is still tagged with the _ngcontent-c0
property which is applied to all template elements on the application root component.
But now, the elements inside the blue-button
template now get applied the _ngcontent-c1
property instead!
Summary of how the host and template element properties work
Let's then summarize how these special HTML properties work, and then see how they enable style isolation:
-
upon application startup (or at build-time with AOT), each component will have a unique property attached to the host element, depending on the order in which the components are processed:
_nghost-c0
,_nghost-c1
, etc. -
together with that, each element inside each component template will also get applied a property that is unique to that particular component:
_ngcontent-c0
,_ngcontent-c1
, etc.
This is all transparent and done under the hood for us.
How do these properties enable view encapsulation?
The presence of these properties could allow us to write manually CSS styles which are much more targetted than just the simple styles that we have on our template.
For example, if we want to scope the blue color to the blue-button
component only, we could write manually the following style:
While style 1 was applicable to any element with the blue-button
class anywhere on the page, style 2 will only work for elements that have that strangely named property!
So this means that style 2 is effectively scoped to only elements of the blue-button
component template, and will not affect any other elements of the page.
So we now can see how those two special properties do enable some sort of style isolation, but it would be cumbersome to have to use those properties manually in our CSS (and in fact, we should not).
But luckily, we don't have to. Angular will do that automatically for us.
How does Angular encapsulate styles?
At startup time (or at build time if using AOT), Angular will see what styles are associated with which components, via the styles
or styleUrls
component properties.
Angular will then take those styles and apply them transparently the corresponding isolating properties, and will then inject the styles directly into the page header as a style
tag:
The _ngcontent-c1
property is unique to elements of the blue-button
template, so the style will be scoped to those elements only.
And that is how the Angular default view encapsulation mechanism works!
This mechanism is not 100% bullet-proof as it does not guarantee perfect isolation, but in practice, it will nearly always work.
The mechanism it's not based on the shadow DOM but instead in these special HTML properties, so if we really wanted to we could still override these styles.
But given that the native Shadow Dom isolation mechanism is currently available only in Chrome and Opera, we cannot yet rely on it.
This mechanism is very useful because it enables us to write simple styles that will not break easily, but we might want to break this isolation selectively from time to time.
Let's learn a couple of ways of doing that, and why we would want to do that.
The :host
pseudo-class selector
Sometimes we want to style the component custom HTML element itself, and not something inside its template.
Let's say for example that we want to style the app-root
component itself, by adding it, for example, an extra border.
We cannot do that using styles inside its app.component.css
associated file, right?
This is because all styles inside that file will be scoped to elements of the template, and not the outer app-root
element itself.
If we want to style the host element of the component itself, we need the special :host
pseudo-class selector. This is the new version of our app.component.css
that uses it:
This selector will ensure those styles are only targeting the app-root
element. Remember that _nghost-c0
property that we talked about before? This is how it's used to implement the :host
selector at runtime:
The use of the special _nghost-c0
will ensure that those styles are scope only to the app-root
element, because app-root
gets added that property at runtime:
If you would like to see a visual demonstration of the :host
pseudo-class selector in action, have a look at this video:
Combining the :host
selector with other selectors
Notice that the can combine this selector with other selectors, which is something that we have not yet talked about.
This is not specific to this selector, but have a look for example at this selector, where we are styling h2
elements inside the host element:
You could be surprised to find out that this style only applies to the h2
elements inside the app-root
template, but not to the h2 inside the blue-button
component.
To see why, let's have a look at the styles that were generated at runtime:
So we can see that the special scoping property gets applied also to nested selectors, to ensure the style is always scoped to that particular template.
But if we did want to override the styles of all the h2
elements, there is still a way.
The ::ng-deep
pseudo-class selector
If we want our component styles to cascade to all child elements of a component, but not to any other element on the page, we can currently do so using by combining the :host
with the ::ng-deep
selector:
This will generate at runtime a style that looks like this:
So this style will be applied to all h2
elements inside app-root
, but not outside of it as expected.
This combination of selectors is useful for example for applying styles to elements that were passed to the template using ng-content
, have a look at this post for more details.
::ng-deep
, /deep/
and >>>
deprecation
The ::ng-deep
pseudo-class selector also has a couple of aliases: >>>
and /deep/
, and all three are soon to be removed.
The main reason for that is that this mechanism for piercing the style isolation sandbox around a component can potentially encourage bad styling practices.
The situation is still evolving, but right now, ::ng-deep
can be used if needed for certain use cases.
The :host-context
pseudo-class selector
Sometimes, we also want to have a component apply a style to some element outside of it. This does not happen often, but one possible common use case is for theme enabling classes.
For example, let's say that we would like to ship a component with multiple alternative themes. Each theme can be enabled via adding a CSS class to a parent element of the component.
Here is how we could implement this use case using the :host-context
selector:
These themed styles are deactivated by default. In order to activate one theme, we need to add to any parent element of this component one of the theme-activating classes.
For example, this is how we would activate the blue theme:
Have a look at this video to see a visual demo of the host-context
selector in action:
All of this functionality that we saw so far was using plain CSS.
But especially in the case of themes, it would be great to be able to extend the CSS language and for example define the primary color of a theme in a variable, to avoid repetition like we would do in Javascript.
That is one of the many use cases that we can support using a CSS preprocessor.
Angular CLI - Sass, Less and Stylus support
A CSS pre-processor is a program that takes an extended version of CSS, and compiles it down to plain CSS.
The Angular CLI supports all major pre-processors, but the one that seems most commonly used in Angular related projects (such as for example Angular Material) is Sass.
In order to use a Sass file instead of a CSS file, we just need to pass such file to the styleUrls
property of a component:
The CLI will then take this Sass file and convert it to plain CSS on the fly. Actually, we can generate new components using Sass files using this command:
ng new cli-test-project --style=sass
We can also set a global property, so that Sass files are used by default:
ng set defaults.styleExt scss
Demo of some the things we can do with Sass
A pre-processor is a great thing to add to our project, to help us write more maintainable styles. Let's have a look at some of the things that we can do with Sass:
If you have never seen this syntax before, it could look a bit surprising! But here is what is going on, line by line:
- on line 2, we have actually defined a CSS variable! This is a huge feature missing from CSS
- we can define not only colors but numbers or event shorthand combined properties such as:
$my-border: 1px solid red
- on lines 6, 10 and 11 we are using the variable that we just created
- on line 9 we are using a nested style, and making a reference to the parent style using the
&
syntax
And this is just a small sample of what we can do with Sass, just a few very commonly used features. The Angular CLI also has support for global styles, that we can combine with our component-specific view encapsulated styles.
We can add global styles not only for the supported pre-processors, but for plain CSS as well.
Summary
There are a ton of options to style our components, so it's important to know which one to use when and why. Here is a short summary:
- sometimes we want global styles, that are applied to all elements of a page - we can add those to our
angular-cli.json
config - the Angular View encapsulation mechanism allows us to write simpler styles, that are simpler to read and won't interfere with other styles
- The default view encapsulation mechanism will bring the long-term benefit of having much less CSS-related bugs. Using it, we will rarely fall into the situation when we add some CSS that fixes one screen but accidentally break something else - this is a huge plus!
- If we are writing a lot of CSS in our project, we probably want to adopt a methodology for structuring our styles from the beginning, such as for example SMACSS
- At a given point we could consider introducing a pre-processor and use some of its features, for example for defining CSS variables
I hope that this post helps in choosing how to style your components, if you have some questions please let me know in the comments below and I will get back to you.
If you would like to learn more about other advanced Angular Core features, we recommend checking the Angular Core Deep Dive course, where component styling is covered in much more detail.
To get notified when more posts like this come out, I invite you to subscribe to our newsletter:
If you are just getting started learning Angular, have a look at the Angular for Beginners Course:
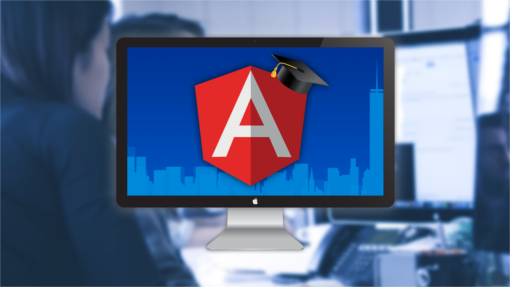
Other posts on Angular
Have also a look also at other popular posts that you might find interesting:
- Getting Started With Angular - Development Environment Best Practices With Yarn, the Angular CLI, Setup an IDE
- Why a Single Page Application, What are the Benefits ? What is a SPA ?
- Angular Smart Components vs Presentation Components: What's the Difference, When to Use Each and Why?
- Angular Router - How To Build a Navigation Menu with Bootstrap 4 and Nested Routes
- Angular Router - Extended Guided Tour, Avoid Common Pitfalls
- Angular Components - The Fundamentals
- How to build Angular apps using Observable Data Services - Pitfalls to avoid
- Introduction to Angular Forms - Template Driven vs Model Driven
- Angular ngFor - Learn all Features including trackBy, why is it not only for Arrays ?
- Angular Universal In Practice - How to build SEO Friendly Single Page Apps with Angular
- How does Angular Change Detection Really Work ?