In this post, we are going to present a series of video tutorials that cover some very commonly used Typescript features.
This video list will help you get a good overview of the language, because some of the features presented here solve several long-term problems that many languages have had during years, such as:
- type safety without verbosity
- fixing the Null problem
- mixed types
More specifically, the videos in this list will cover:
- some commonly used ES6 features supported by Typescript
- Debugging in the Browser And Node
- Typings and Type System Features
Table Of Contents
Here is the list of topics covered in the videos available in this post, each of these topics has a video associated with it plus a description, all videos are part of this Playlist:
- Video 1 - Top 4 Advantages of Typescript - Why Typescript?
- Video 2 - ES6 / Typescript let vs const vs var When To Use Each? Const and Immutability
- Video 3 - Learn ES6 Object Destructuring (in Typescript), Shorthand Object Creation and How They Are Related
- Video 4 - Debugging Typescript in the Browser and a Node Server - Step By Step Instructions
- Video 5 - Build Type Safe Programs Without Classes Using Typescript
- Video 6 - The Typescript Any Type - How Does It Really Work?
- Video 7 - Typescript @types - Installing Type Definitions For 3rd Party Libraries
- Video 8 - Typescript Non-Nullable Types - Avoiding null and undefined Bugs
- Video 9 - Typescript Union and Intersection Types- Interface vs Type Aliases
- Video 10 - Typescript Tuple Types and Arrays Strong Typing
Video 1 - Top 4 Advantages of Typescript - Why Typescript?
The Typescript language has been evolving a lot in the last year, so many features were introduced recently and more are being added with each minor release. With all these features, it's easy to accidentally lose sight of what are the main benefits of using the language to start with.
One common thing that gets mentioned about Typescript is that there is a verbosity price to pay for the type-checking benefits, in a similar way to what happens nominally typed languages such as for example Java or C#.
That is actually not the case, with Typescript we can have both the conciseness and readability together with the tooling benefits. To learn more about the main advantages of the Typescript language, have a look at this video:
Video 2 - ES6 / Typescript let vs const vs var When To Use Each? Const and Immutability
One of the main features of Typescript is benefitting from many ES6+ features without having to wait for generalized browser support.
With Typescript, we can write our programs using all the latest Javascript features, even the ones at earlier stages of adoption. The code will then be transpiled from Typescript to a Javascript version that is currently supported across all browsers.
The ES6 features that are supported is a continuous moving target, but with Typescript that is one less thing to worry about. Using it we can sure that our code will run in all browsers, independently of the level of support of each browser for any given feature.
To have a look at a very commonly used ES6 feature supported by Typescript, have a look at this demo video about the let
vs const
keywords, and learn which one we could be using by default and why:
Video 3 - Learn ES6 Object Destructuring (in Typescript), Shorthand Object Creation and How They Are Related
When learning new ES6+ language features, it's sometimes hard to understand what is the main benefit of the feature, and why it was added to the language.
This is because many times the multiple features are designed to be used together, and we will make the most sense of them if we see them in an example where multiple features are combined together.
A good example of this are the following features:
- Object Destructuring
- Shorthand Object Creation
Each of these features does not seem so important if seen isolation, but have a look at this video to see how they really allow us to write more concise programs when used together:
Video 4 - Debugging Typescript in the Browser and a Node Server - Step By Step Instructions
Sometimes we see that to debug Typescript we often need to debug the transpiled Javascript, but that has not been the case now for a while.
There is currently great support for debugging Typescript directly in the browser, in IDEs like Webstorm, and this also includes the debugging of Node programs written in Typescript.
To have a look at how we can debug Typescript in both Node and the browser, have a look at this video with a demo:
Video 5 - Build Type Safe Programs Without Classes Using Typescript
Going beyond primitive types, a very common misconception about Typescript is that we have to use object-oriented constructs in order to have custom object types in our program, and that is actually not the case.
We can define our own object types without using classes, by using the interface
keyword. This keyword, unlike in other languages, is not only an object-oriented construct; in Typescript, interface
doubles as a way of defining custom object types.
To understand better how the type system works under the hood (it's very different from for example Java or C#), have a look at this post, but if you would like to see what the use of the interface
keyword looks like while defining custom object types, have a look at this video:
Note: There is more on this topic also on video 9
Video 6 - The Typescript Any Type - How Does It Really Work?
One of the key features of the Typescript language is its out-of-the-box integration with pure Javascript.
If we take an existing Javascript codebase and we apply it the Typescript compiler, we should have something very close to a compiling program.
We might have to change a couple of compiler flags and add some type annotations depending how far we want type safety to work, but in general, we should be able to migrate the codebase quickly.
And a key language feature for that level of compatibility is the Typescript any
type. To learn more about the Typescript any
type and how we can use it in our programs, have a look at this demo video:
Video 7 - Typescript @types - Installing Type Definitions For 3rd Party Libraries
One of the most useful features of the Typescript language are type definitions: but what do when using libraries that don't provide typings?
If a library provides their own typings inside their npm package then it's better to use those. This is the case for example for Firebase, and more commonly used packages are starting to provide their own type definitions.
But if we are using a common package written in plain Javascript with no type definitions like Express, we can many times stiff benefit from type-safety by installing a third-party package that contains only the type definitions.
To learn more about how to obtain third party type definitions, have a look at this video on the npm @types
packages:
Video 8 - Typescript Non-Nullable Types - Avoiding null and undefined Bugs
This is one of the most sought features in many languages (have a look at this post Null References: The Billion Dollar Mistake): the ability to write a program and be sure that we will not run into null / undefined issues.
This is now possible with Typescript, if we turn on a couple of compiler flags. These are off by default because existing codebases would give a lot of errors if compiled using this feature, so it would be a big breaking change if they were on by default.
To see this feature in action, and see how we can opt-in to these stronger type checkign mechanism, have a look at this video:
Video 9 - Typescript Union and Intersection Types- Interface vs Type Aliases
On video 5 we saw how to use the interface
keyword for defining object types. There is another closely related keyword with is the type
keyword, that is meant for combining multiple type definitions together.
This is especially useful if combined with strict null and undefined checking presented on the previous video, because it allows us to define a combined type that can be either an instance of an object or null/undefined.
There are also other cases where it's useful to able to combine types, because sometimes we run into these more advanced use cases:
- we want to have a function that takes in for example either an object or an array as a configuration element
- we want to have a function return different types, depending on its inputs
In those cases we can use Typescript union and intersection types to express those use cases, instead of resorting to the any
keyword. In order to see this functionality in action, have a look at this video:
Video 10 - Typescript Tuple Types and Arrays Strong Typing
There are some very common use cases that we usually run into, and for which no non-verbose solution exists in many other languages.
Sometimes we want to return a couple of data structures or related values from a method, but we don't necessarily want to have to define a custom type for that.
In other occasions, we would like to limit the length of an array defined as part of an input or output type. We would like to, for example, specify that an array should have 3 elements.
These common use cases are well supported by Typescript via Typescript Tuples. To see what this looks like in practice, have a look at tuple types in action in the following video:
Related Posts
If based on this list you would like to go deeper into the multiple Typescript type definitions and see when to use each and why, have a look this post:
If you would like to learn more about the type system itself and see what is the fundamental difference towards nominal type systems (like Java or C#), have a look at this post:
I hope you enjoyed the post, I invite you to have a look at the list below for other similar posts and resources on Angular.
I invite you to subscribe to our newsletter to get notified when more posts like this come out:
If you are just getting started learning Angular, have a look at the Angular for Beginners Course:
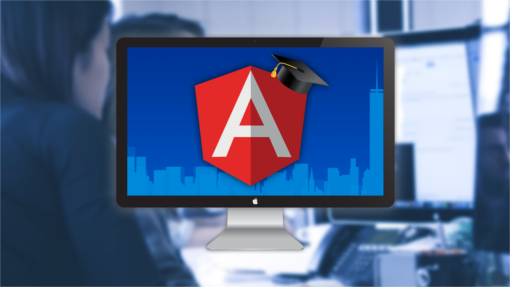
Other posts on Angular
If you enjoyed this post, have also a look also at other popular posts that you might find interesting:
- Angular Router - How To Build a Navigation Menu with Bootstrap 4 and Nested Routes
- Angular Router - Extended Guided Tour, Avoid Common Pitfalls
- Angular Components - The Fundamentals
- How to build Angular apps using Observable Data Services - Pitfalls to avoid
- Introduction to Angular Forms - Template Driven vs Model Driven
- Angular ngFor - Learn all Features including trackBy, why is it not only for Arrays ?
- Angular Universal In Practice - How to build SEO Friendly Single Page Apps with Angular
- How does Angular Change Detection Really Work ?
- Typescript 2 Type Definitions Crash Course - Types and Npm, how are they linked ? @types, Compiler Opt-In Types: When To Use Each and Why ?