If you are trying to learn Angular as a beginner, or if you are already experienced and want to take your knowledge to the next level, you probably have already asked yourself what is the best way to learn Angular.
We have been teaching thousands of Angular developers throughout the years and we have received a lot of feedback about the most common learning pain points.
In this post, we have compiled what we think is the best way of learning Angular, in order to quickly get proficient with it.
We are also going to provide multiple alternative paths for learning in depth the Angular ecosystem, so that you can choose what suits you best.
We will also propose a couple of different backend technologies, that we think that make an especially good fit for Angular frontends.
Table Of Contents
In this post, we will discuss the following topics:
- Angular For Beginners
- Angular Core Deep Dive
- RxJs In Practice
- Angular Forms In Depth
- Angular Router In Depth
- Reactive Design Patterns
- NgRx and NgRx Data
- Angular Testing
- Angular Material
- Angular Security
- Angular Universal
- Progressive Web Apps
- REST with NestJs and MongoDB
- Firebase & AngularFire
- Online Payments
Angular For Beginners
There is a lot to learn about Angular, and one of the main dangers for a beginner is to get overwhelmed and to not even know where to start!
We recommend starting by learning only the most important and also most commonly used parts of the core framework, which includes:
- Components
- Input, Outputs, Events
- Core Directives; ngFor, ngIf, etc.
- Component Styling, ngClass, ngStyle, etc.
- Pipes
- Basics of dependency injection
- Services
- HTTP Client (GET, POST, PUT, DELETE)
- Modules
Focusing on much more than this at this stage could easily become counterproductive, so we recommend focusing on these essential Angular notions first before moving on to something else.
In practice, the content of this course is already a lot of what you will need to use to build Angular applications.
The Angular Beginners Course is an over 2 hours free course that is aimed at getting a complete beginner in Angular comfortable with the main notions of the core parts of the framework:
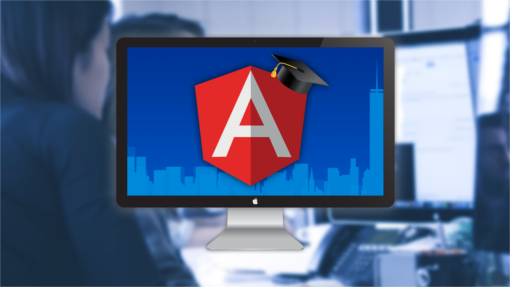
Notice that this course, like all courses in this list has available a working Github repository, available in the Angular University Github page.
These github repositories have in the master branch the completed solution of all the exercises in the course, under the form of a sample Angular application.
Each course also has a starting branch usually called 1-start
, that you can use as a clean starting point for coding along throughout the course.
Notice that coding along is not mandatory for taking any of the courses. Each course will still make perfect sense if you take them by watching them for example on a tablet in case that you don't have a desktop handy.
But we recommend if possible to code along and try to implement all the use cases yourself, as this will speed up your learning process.
Angular Core Deep Dive
After the beginners course, we already get to our first branching point in your Angular learning path.
If you went through the beginners course quickly, you might already be prepared for something more advanced.
If you want to learn right from the start all the advanced features that the Angular Core module bring us, then you can take some time to learn the following features:
- Lifecycle Hooks (ngOnInit, ngOnDestroy, etc.)
- @ViewChild and AfterViewInit
- @ViewChildren
- ng-content and Component Projection
- @ContentChild and AfterContentInit
- @ContentChildren
- ng-template & ng-container
- ngTemplateOutlet
- Change Detection (default, OnPush and custom)
- View Encapsulation modes
- Custom Directives
- Structural Directives
- Dependency Injection in detail
- advanced styling options: host, host-context, etc.
- @NgPlural and other i18n features
- Angular Elements
The Angular Core Deep Dive is an 8.5 hours course that covers in detail all of these features available in the Angular core module:
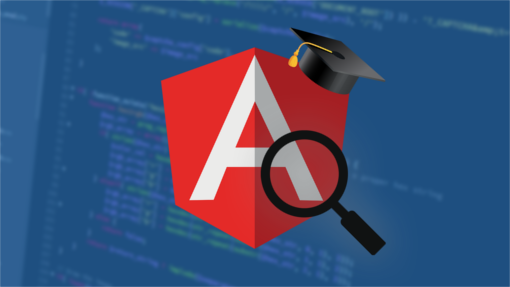
Notice that the beginners course is a subset of the Angular Core deep dive.
However, if you struggled a bit with the beginners course, it might be too soon for getting into these features at this stage.
Instead, it would be a lot more productive for you to go back to the beginners course and really nail those essential core features down, and then skip for the moment the Angular Core Deep dive course and instead focus on learning RxJs and Observables.
This will be much more important in practice in terms of day-to-day application development, and a much better use of your learning time.
You can always come back to these more advanced core features later when you feel ready.
RxJs In Practice
In order to become proficient as an Angular application developer, much more important than knowing every single advanced feature of the Angular core module, is to have a solid practical understanding of Observables and the most commonly used RxJs operators.
RxJs is used in many places in the Angular core API, and its also the recommended way for building the application service layer.
Some of the most common application development tasks like doing HTTP requests revolve around the notion of Observables, so you will have to have a good understanting of them.
Although its very tempting at the beginning, we think that its counterproductive to focus on trying to learn too many RxJs operators.
We think that its much more important instead to have a solid understanding of the notions of:
- Stream
- Observable
- Subscriptions
- The Observable contract
- learn how to read marble diagrams
With these notions in place, then we can focus on learning the most commonly used 15 to 20 RxJS operators, that in practice are all that we will need for implementing the vast majority of use cases.
If we ever run into a very particular use case, we can always learn that extra RxJs operator that we need, on a case by case basis.
In order to help you learn RxJs in what we believe is the most effective way, you have available the RxJs In Practice Course:
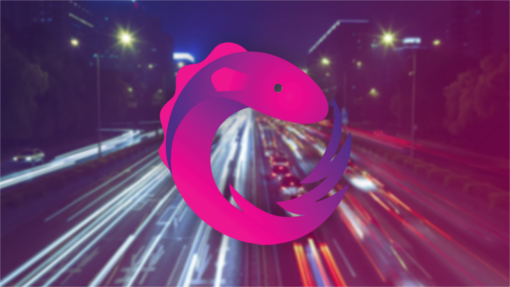
This course takes 4.5h, and it will help you a lot to boostrap your RxJs knowledge. We will start with the beginning introducing the notions of stream, Observable, the Observable contract, etc.
You will learn how to read marble diagrams, and you will use them to learn a series of very commonly used RxJs operators.
We will also cover more advanced RxJs concepts like subjects, custom observables, and more.
Angular Forms In Depth
After getting a good understanding of Angular core and RxJs, we recommend that you learn next the Angular Forms module.
Angular is used a lot to build large form-intensive business applications, which typically have large forms with complex business validation rules.
One of the mains reasons why Angular is so well suited for that type of aplications is the Angular forms module, that makes it very simple to build complex forms in a maintainable way.
You have two ways of building forms: template-driven and reactive forms, but its recommended to use reactive forms for new applications.
You can read more about the reasons why reactive forms are in general preferable to template driven.
As template-driven forms are also very popular, for completeness, you can learn about both form types in detail in the Angular Forms In Depth course:

In this course, you will build a form in both template driven and reactive style in order to compare the two form types, and you will also build a larger multi-step form with all sorts of custom validators.
You will learn all about all sorts of advanced form topics, including custom form controls, embedded form groups, form arrays and much more.
Angular Router In Depth
After the Forms module, which you will use a lot of time to build many of the screens of your application, you want to think about the application navigation system.
As you know, Angular is meant to build single page applications, which are large Javascript-heavy applications that take over the navigation system of the page and don't need full server page payloads to transfer between application pages.
Instead, the user remains in the initial HTML page (which explains the name single page application), and only certain sections of the page are adapted depending on a given navigation action.
The Angular Router is a very powerful Angular module that allows us to decide which components should be displayed to the user depending on the value of the url.
With the Angular Router In Depth course (5 hours) you will learn everything that you need to know for building the navigation system of your application using the Angular Router:

All sorts of advanced routing topics are covered in the course, including a master-detail with detail-to-detail navigation scenario, lazy loaded routes, secondary router outlets and much more.
Reactive Design Patterns
Once you start building your Angular application, with already a good understanding of the core, forms, and router modules as well as RxJs, you will notice that a whole different type of questions start coming up.
Yes, you know how the individual modules and features work at this point, but the problem is, how to tie everything together?
How are these multiple pieces of the application puzzle meant to be used together, how to combine the multiple concepts in order to implement common use cases?
The most common way of building Angular applications is in reactive style, by relying a lot on RxJs to integrate the view and service layer.
What we need at this point in your Angular learning journey is a series of commonly used design patterns (or application design recipes) that will help you implement common use cases in reactive style.
This includes:
- how to handle application state with plain RxJs
- Smart vs Presentational Components
- Stateless Observable Services
- Decoupled component communication using shared observable services
- Error Handling and error messages in reactive style
- Loading Indicators in reactive style
- lightweight state management RxJs stores
- Optimistic UI updates
- Managing User Authentication state with plain RxJs
- Master Detail with cached master table in reactive style
- The Single Data Observable Pattern
The Reactive Angular Course (5.5h) covers these patterns and many more:

NgRx and NgRx Data
We think that it's important to first try to develop your application using plain RxJs only, using some of the design patterns covered on the reactive patterns course.
If you then run into state management problems, that can't be easilly solved using only plain RxJs, only then do we recommend considering a state management solution.
The most commonly used state management solution in the Angular ecosystem is NgRx.
NgRx is a RxJs-based implementation of the Redux centralized store pattern, and is based upon the concepts of actions, reducers, selectors and effects.
NgRx comes with a companion library called NgRx Data, which is meant to make it much easier to do state management of entity-like data.
Notice that NgRx Data is not meant to replace plain NgRx techniques, but instead complement them.
The NgRx (with NgRx Data) - The Complete Guide course (6h) covers both Ngrx and NgRx Data in detail:

Angular Testing
Before getting started in a project as an Angular developer, we think that it's really essential to learn in detail how to write Angular tests.
Depending on the company that you work on, you might expect to maintain not only the application main code base but also large test suites that go along with it.
Angular Unit Testing requires the developer to have a good knowledge of how to manually configure the Angular dependency injection system in order to mock component dependencies, as well a good notions on how change detection works, as well other more advanced concepts.
Besides unit testing, we can also create integration tests that test how a component interacts with other components and services in a production-like scenario.
The Angular Testing Course (5h) will teach you everything that you need to know in order to feel comfortable writing and troubleshooting Angular Unit Tests using the Jasmine framework and integration tests with the Cypress end-to end testing framework:
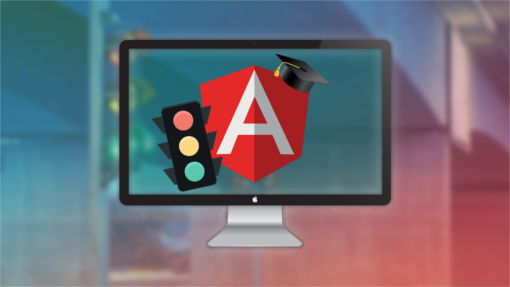
Angular Material
The Angular Material library contains a series of very powerful and customizable components that are ideal for building Angular applications using the Material design framework.
Knowing Angular Material is not mandatory, especially if you are not building a new application from scratch, or if you are not planning on using Angular Material by some reason.
But think twice, because by using Angular Material, you will never have to spend time again implementing your own datepicker, dropdown box, and many other commonly needed components that you will need in any application.
Also, the Angular Material components already look great and they come with a pre-defined set of themes that makes it very simple to build a great looking user interface with much less custom CSS development than you would otherwise have to do if implementing all the styling in your application from scratch.
If you want to learn in detail all about the multiple components available in Angular Material, check out the Angular Material In Depth course:

Angular Security
If you are planning on evolving to architecture level positions, then the more advanced topics covered in the next series of courses will become more important.
On of those more advanced topics is definitively security: how do you make sure that your Angular application are as secure as possible?
Web Security includes many different sub-topics, so in the Angular Security Course (8h), we will focus mostly on Authentication and Authorization using JWTs (JSON Web Tokens):
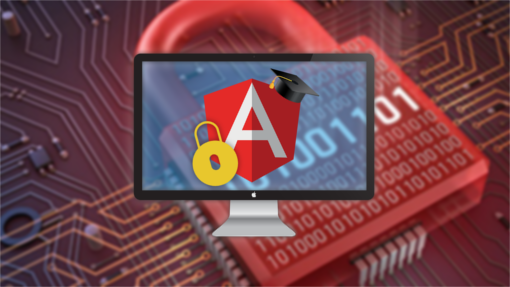
We are going to build (for learning purposes only) our own authentication system from scratch, including safe storage of passwords in a database, and later we are going the refactor our solution to use the Auth0 third-party authentication system.
We will also learn how to do RBAC (Role Based Access Control) Authorization with JWTs. Our application is going to use Angular for the frontend, and Node for the backend.
Even though the backend is built specifically in Node, all the backend security concepts discussed using Node are also applicable to other backends as well, such as PHP, Java, C#, etc.
Angular Universal
Another advanced topic that you might want to look into is Angular Universal or server-side rendering in general.
This is especially true if you are developing a public-facing application that is going to be live on the public internet, and that you would like to have indexed by search engines.
Angular Universal helps with search engine optimization and also offers an improved application startup time. Actually, performance startup and showing some content to the user as soon as possible might be the most important reason for which you would want to use Angular Universal.
In the Angular Universal In Depth course, we will cover everything that you need to know in order to server-side render your application, or even pre-render it at build time:

Progressive Web Apps
Another advanced topic that you might need to learn about are PWAs or Progressive Web Applications. These are standard web applications that have the ability to download themselves in the background to the user phone or desktop, just like a mobile application.
In the Angular PWA Course we are going to take an existing Angular sample application, and we are going to turn it into a PWA using the Angular Service Worker.
We will learn in detail how the service worker lifecycle works, by building our own service worker from scratch:
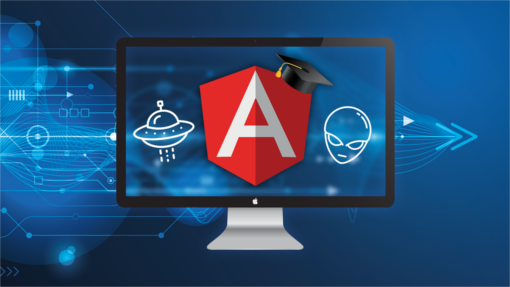
REST with NestJs and MongoDB
The next couple of topics that we will be discussing are backend-related. We are going to present some of the available backend solutions that we think work especially well when used together with Angular.
If you are planning on building the backend of your application also in Typescript, then you might want to look into the NestJS framework.
The NestJs framework is especially useful for Angular developers, because many of the concepts that we use to build the Angular frontend (like services, dependency injection, pipes) are also used on the backend.
With NestJs, you can build your REST backend using very similar design principles and using the same language as you already use to build your Angular frontend.
In the NestJs In Practice course, we are going to learn how to build a REST backend using NestJs that accesses a MongoDB database:

Firebase, Firestore and AngularFire
If instead of manually building your own REST end points, you would prefer to go for a serverless solution with a managed out-of-the-box backend, then we recommend checking the Firebase ecosystem.
Using Firestore, you can even do not only your data retrieval queries, but also your data modification operations straight from the Angular client and into the Firestore server, in a secure way.
Security is ensured in a declarative way via Firestore Security rules, which allow you to enforce the authentication and authorization of your Angular client requests in a granular way, without the need to run your own backend.
The Firebase ecosystem is complete with a solution for Authentication called Firebase Authentication, that allows you to authenticate users using many third-party authentication providers such as Google, Facebook, etc., as well using plain email and password.
You also have a secure file upload solution named Firebase Storage, and you can deploy your own managed backend server and database triggers using Firebase Cloud Functions.
In the Firebase & AngularFire In Depth course, we will cover in detail all of these aspects of the Firebase ecosystem:

Online Payments
We wanted to give you a complete solution for how to build a fully functional web application, and we felt that online payments was the missing piece.
Although this course is not essential if you are not working on an application that does online payments, this might get you on the way to build your own prototype for a new business application that you might want to develop outside of work.
Or, if you are planning on doing the transition into startup-based jobs, then knowing how to handle online payments is a great advantage.
In the Stripe Payments in Practice course, you will learn how to add payments to an existing Angular application using Stripe and Firebase (with the Firestore database) as the backend:

Besides teaching online payments (both one-time and recurring charges), this course is also a great end-to-end application building exercise that ties together many of the things that we learned in the previous courses, such as web security, authentication and Firebase.
Summary
Here is the best way to learn Angular:
- start with the Angular for Beginners course, and master the major core concepts that you will be using all the time, like components, services, modules, etc.
- learn the Angular core features if you want early on, but bear in mind that some of these features are less frequently used at the application level, so you might want to learn them later instead, its up to you
- Focus on learning the main concepts of RxJs, like the notions of stream, Observable and the Observable contract, as well as the most commonly used operators.
- Don't invest a lot of time learning all the RxJs operators available, as this can quickly become counterproductive
- learn early on the Forms module, as its one of the modules that you will be using more often, and focus on reactive forms as opposed to template driven forms
- Learn how to setup the navigation system of your application using the Angular router
- Learn how to design both the view and the service layer of your application using simple RxJs techniques, including lightweight state management
- only if of those techniques are not sufficient, then consider learning a state management library like NgRx
- NgRx comes with NgRx Data, which is meant to handle entity state. This is meant to be used as a complement to standard NgRx techniques, and not replace them
- Learn more advanced topics only as you need them or if you want to improve your knowledge for the future: for example security, server-side rendering or progressive web apps are examples of topics that are not essential at the beginning but that later on you might be curious to learn more about
- use as much as possible the Angular Material library, to avoid having to implement your own widgets from scratch, or having to use third-party jQuery based widgets that will increase a lot the Javascript payload of your page
- If you are looking to build your backend also in Typescript, you might want to have a look at NestJs, which is a Typescript-based web framework that reuses many of the concepts that we use also while building Angular frontends, like components, services, modules, etc.
- If you are looking for a serverless option for your backend, the Firebase ecosystem and especially the Firestore database provide a great solution. These are especially well integrated with Angular via the AngularFire library
You can find all the courses listed in this post in the Angular University website home page, ordered by the recommended learning order.
I hope you enjoyed the post, I invite you to have a look at the list below for other similar posts and resources on Angular.
I invite you to subscribe to our newsletter to get notified when more posts like this come out:
If you are just getting started learning Angular, have a look at the Angular for Beginners Course:
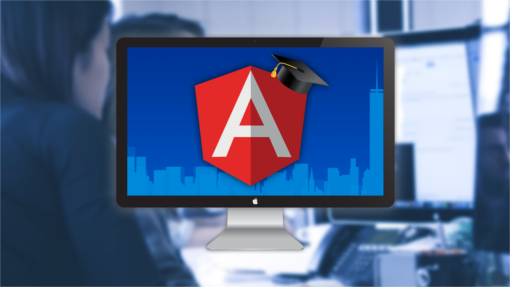
Other posts on Angular
If you enjoyed this post, have also a look also at other popular posts that you might find interesting:
- Angular Router - How To Build a Navigation Menu with Bootstrap 4 and Nested Routes
- Angular Router - Extended Guided Tour, Avoid Common Pitfalls
- Angular Components - The Fundamentals
- How to build Angular apps using Observable Data Services - Pitfalls to avoid
- Introduction to Angular Forms - Template Driven vs Model Driven
- Angular ngFor - Learn all Features including trackBy, why is it not only for Arrays ?
- Angular Universal In Practice - How to build SEO Friendly Single Page Apps with Angular
- How does Angular Change Detection Really Work ?
- Typescript 2 Type Definitions Crash Course - Types and Npm, how are they linked ? @types, Compiler Opt-In Types: When To Use Each and Why ?